Tile
Tile is surface that display content and actions on a single topic. It should be easy to scan for relevant and actionable information. Elements, like text, images or buttons, should be placed on Tile in a way that clearly indicates hierarchy.
Examples
Default
The default Tile comes with a three mandatory props, img
, title
and a text
. The following optional props are: as
(polymorphic prop), href
, hero
(object), hasShadow
, children
, childrenBeforeText
, footer
, onFavoriteClick
.
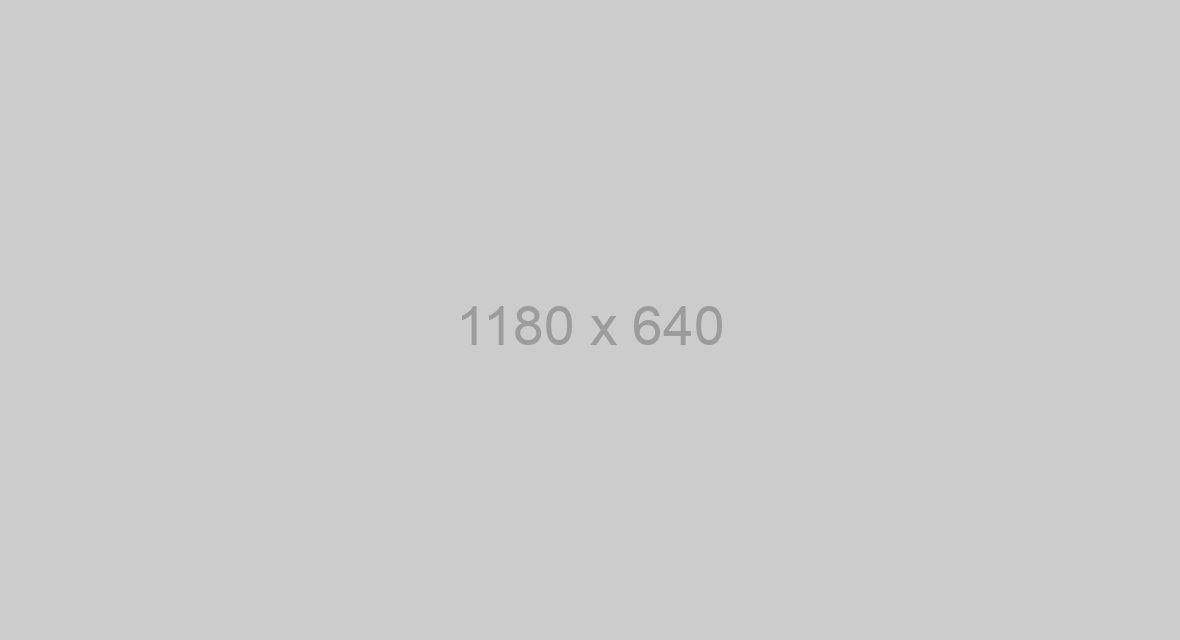
What makes an espresso good?
A good espresso is the result of several factors coming together harmoniously. These factors can be broken down into beans, equipment, and technique.
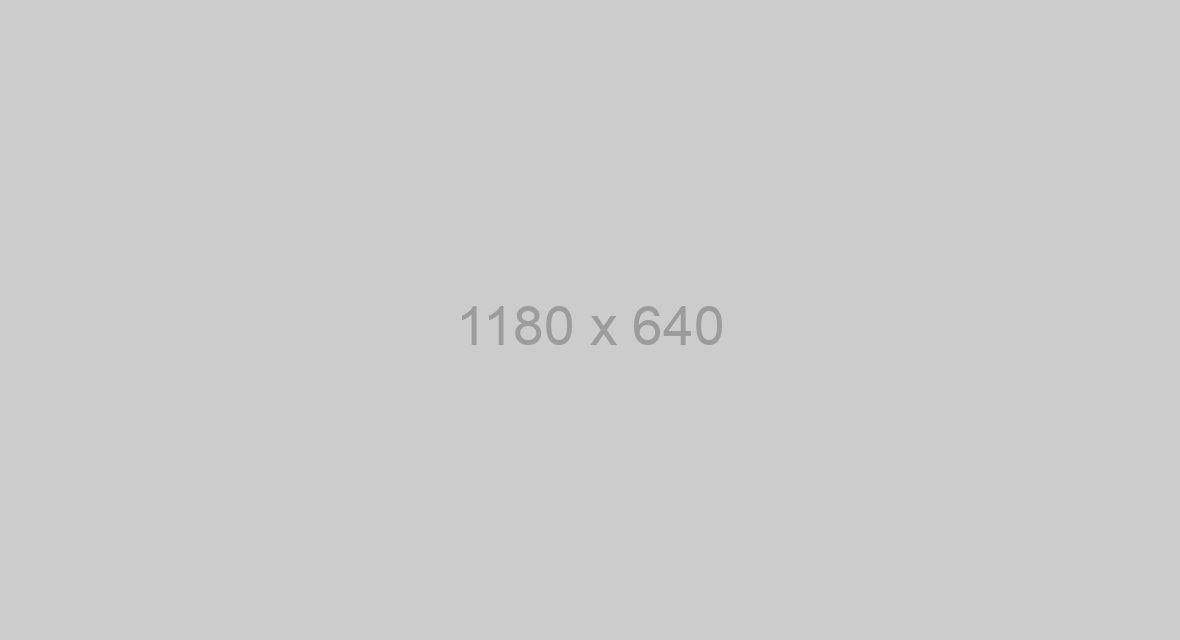
What makes an espresso good?
A good espresso is the result of several factors coming together harmoniously. These factors can be broken down into beans, equipment, and technique.
import { Tile } from '@millanbrankovic/styled-ui-library';
function DefaultTileExample() {
return (
<Tile
img={{ src: 'https://picsum.photos/id/63/1180/640' }}
title={{ title: 'What makes an espresso good?' }}
text={{ title: 'A good espresso is the result of several...' }}
/>
);
};
export default DefaultTileExample;
Appearance
Hero
When the hero
prop is provided, it renders an overlay on the image and necessitates the inclusion of the title
prop. Both label
and action
props are optional. The action
prop accepts a type of React.ReactNode
. In this instance, a Button
is utilized. Also, in this example, since onFavoriteClick
is passed, it automatically displays a 'heart' icon (ButtonIcon
component).
New Espresso machine is here!
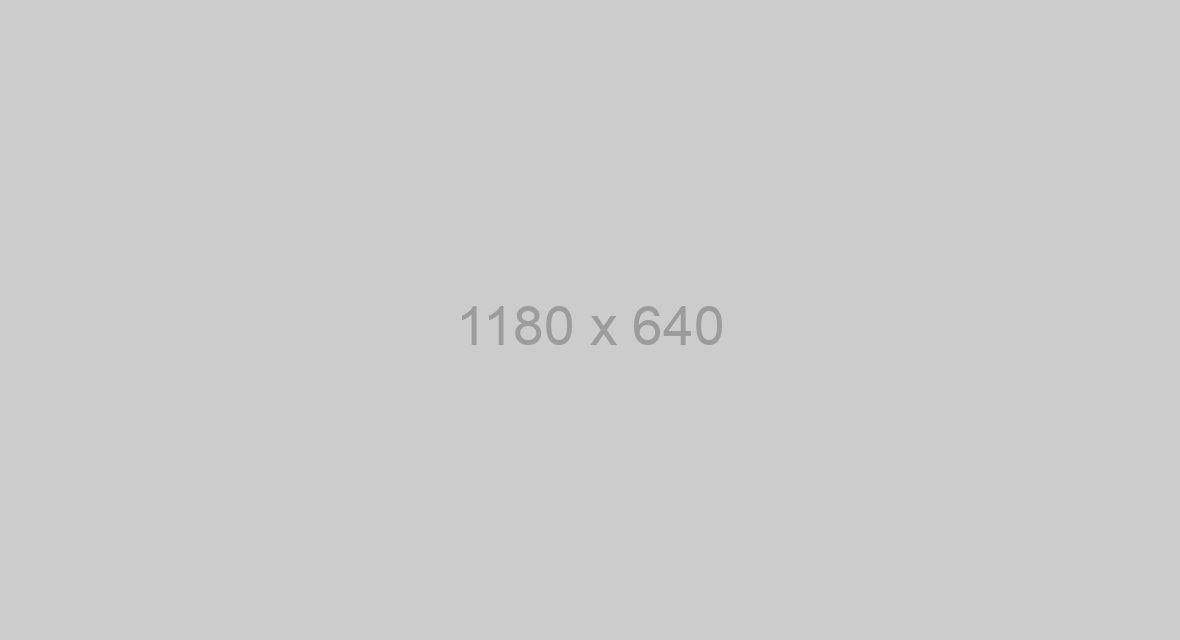
JURA E8 new generation
What makes the newly designed JURA E8 fully automatic coffee machine so convincing. The E8 fully automatic machine stands out with its very well thought-out features.
New Espresso machine is here!
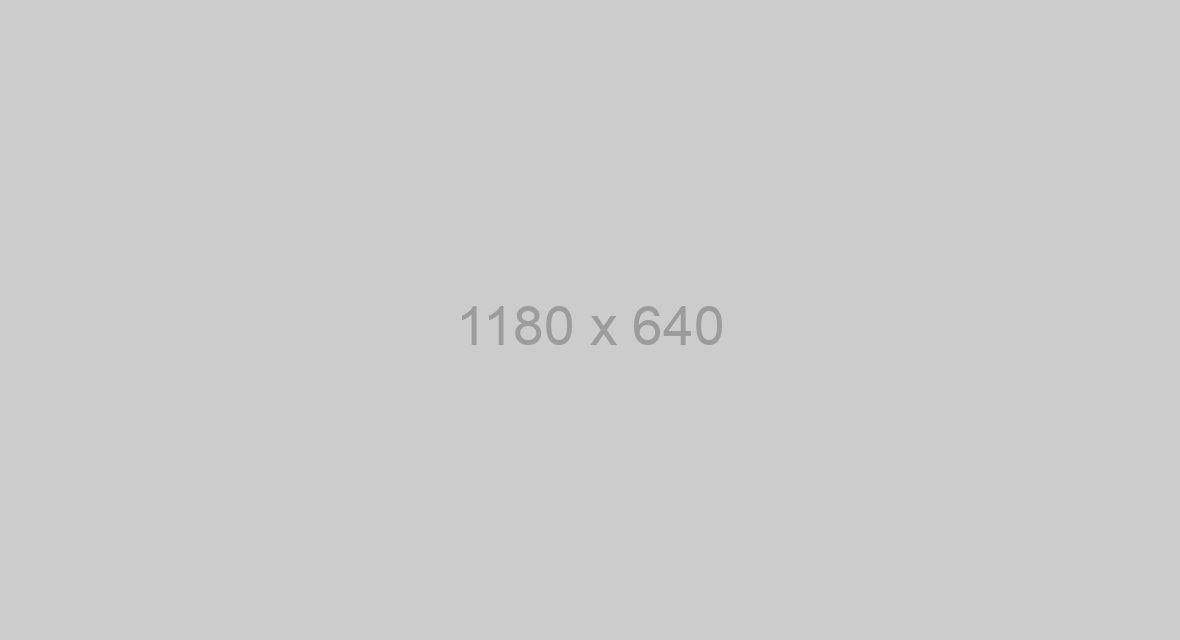
JURA E8 new generation
What makes the newly designed JURA E8 fully automatic coffee machine so convincing. The E8 fully automatic machine stands out with its very well thought-out features.
import { Tile } from '@millanbrankovic/styled-ui-library';
function HeroTileExample() {
return (
<Tile
img={{ src: 'https://picsum.photos/id/63/1180/640' }}
title={{ title: 'JURA E8 new generation' }}
text={{ title: 'What makes the newly designed JURA E8...' }}
hero={{
title: { title: 'New Espresso machine is here!' }
label: { title: 'Pre-order' }
action: <Button title="Find out more" appearance="primary" isRound />
}}
onFavoriteClick={() => alert('Added to favorites!')}
/>
);
};
export default HeroTileExample;
Children
A children
prop is an optional prop which can be used to render the additional content. It accepts any content type. In conjunction with children
, an additional prop childrenBeforeText
(boolean) can be used to render the children's content above the default text. In this example, as
and href
props are used which transforms the entire Tile
into a link.
import { Tile } from '@millanbrankovic/styled-ui-library';
function ChildrenTileExample() {
return (
<Tile
as='a'
href='#'
img={{ src: 'https://picsum.photos/id/63/1180/640' }}
title={{ title: 'What makes an espresso good?' }}
text={{ title: 'A good espresso is the result of several...' }}
children={
<>
<Text
title="Children example:"
weight="bolder"
marginBottom={{ xs: 8, md: 8, lg: 8 }}
/>
<List isOrdered>{renderList}</List>
</>
}
/>
);
};
export default ChildrenTileExample;
A footer
prop also sometimes comes in handy and it can render anything. In this example, it renders an array of Chip
components, but it can also be a string or any other single component or multiple components.
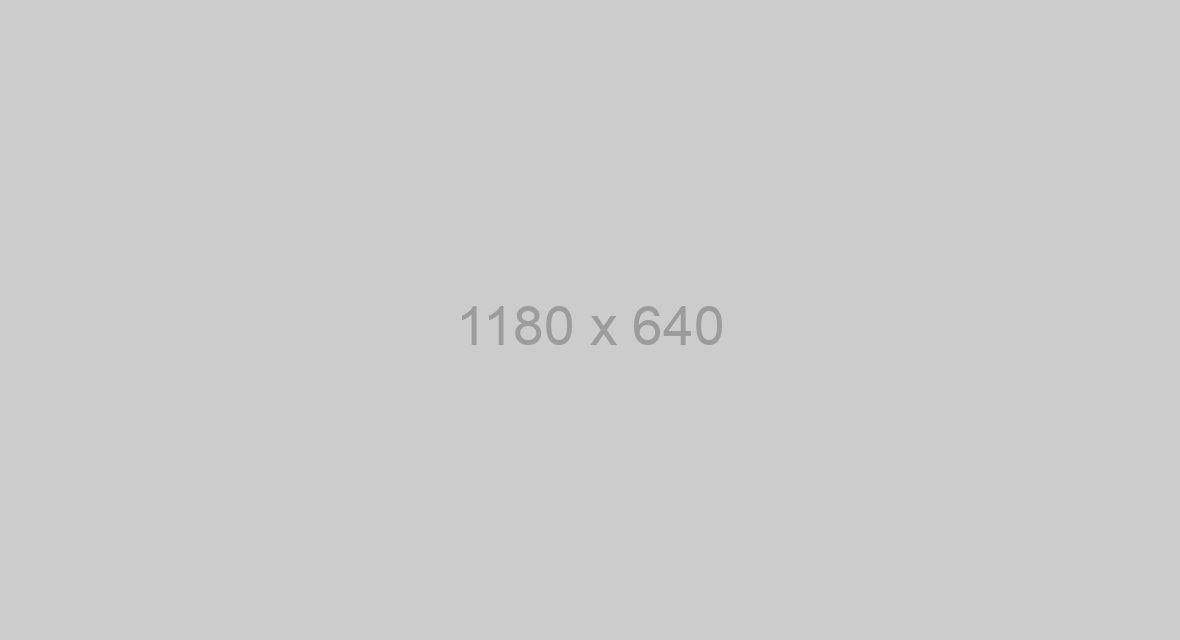
What makes an espresso good?
A good espresso is the result of several factors coming together harmoniously. These factors can be broken down into beans, equipment, and technique.
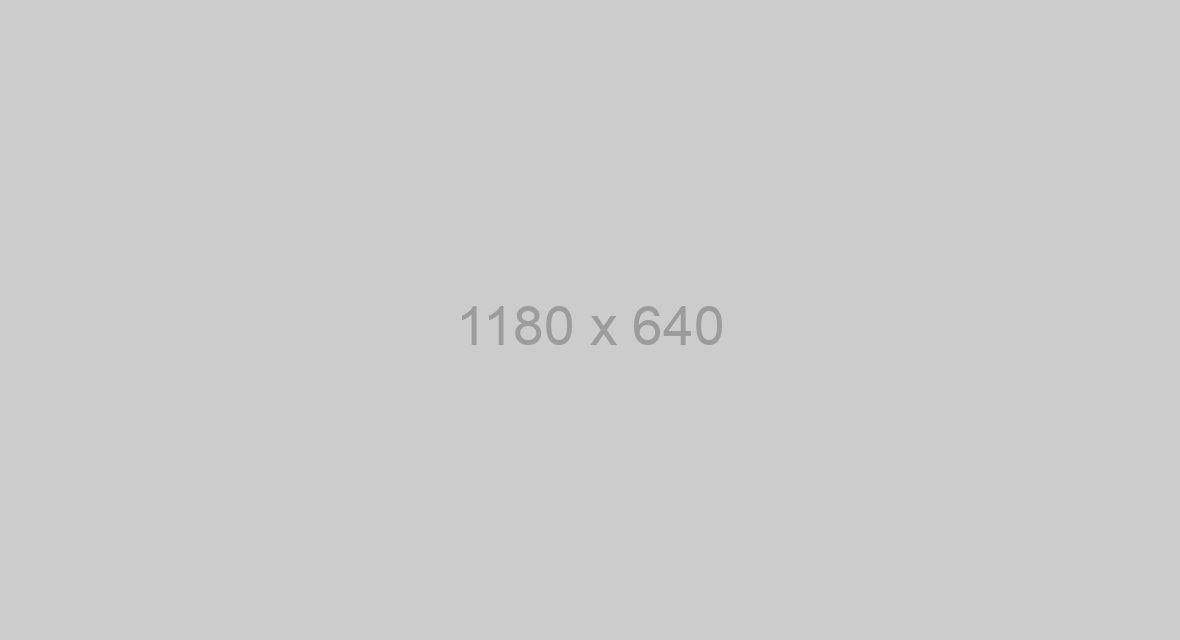
What makes an espresso good?
A good espresso is the result of several factors coming together harmoniously. These factors can be broken down into beans, equipment, and technique.
import { Tile } from '@millanbrankovic/styled-ui-library';
function FooterTileExample() {
return (
<Tile
img={{ src: 'https://picsum.photos/id/63/1180/640' }}
title={{ title: 'What makes an espresso good?' }}
text={{ title: 'A good espresso is the result of several...' }}
footer={
<>
<Chip label="Food" size="small" />
<Chip label="Health" size="small" />
<Chip label="Recipes" size="small" />
<Chip label="Coffee" size="small" />
</>
}
onFavoriteClick={() => alert('Added to favorites!')}
/>
);
};
export default FooterTileExample;